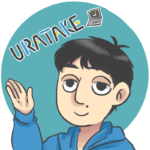
今回はif文について解説をさせていただきます。
これまで出てきたデータ型や演算子を使いながらご紹介をさせていただきます。
if文が使いこなせるとプログラミングをやってる感が出ますよ!
制御構文とは
順次(記述された順番に実行する)、選択(条件によって処理を分岐する)、反復(特定の処理を繰り返し実行する)を
組み合わせてプログラムを作成します。これらの制御構文について解説をさせていただきます。
選択とは
特定の条件に応じて処理を分岐させる構文となります。
If文というと一度は聞いたことがある方がいらっしゃると思います。
Pythonでは以下のように記述します。
if 条件式:
条件式がTrueの場合の処理
else:
条件式がFalseの場合の処理
そろそろ実際のプログラムを書いてみて紹介をしてみようと思います。
今回作るのはじゃんけんのプログラムにします。
仕様としてはこんな感じです。
- じゃんけんの手を数字で入力してCPUと対戦する。
- じゃんけんした結果が「勝ち」「あいこ」「負け」のいずれかが出力される。
- 想定していない数が入力されたら「無効試合」と出力される。
実際のプログラムはこんな感じ
import random
cpu_hand = random.randint(0,2)
player_hand = input('じゃんけんの手を入力してください:(0=グー、1=チョキ、2=パー)')
player_hand = int(player_hand)
if cpu_hand == 0:
cpu_result = 'グー'
elif cpu_hand == 1:
cpu_result = 'チョキ'
else:
cpu_result = 'パー'
if player_hand == 0:
player_result = 'グー'
elif player_hand == 1:
player_result = 'チョキ'
else:
player_result = 'パー'
if player_hand >= 3:
print('無効試合')
elif player_hand == cpu_hand:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あいこ')
elif player_hand - cpu_hand == 2:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの勝ち')
elif player_hand - cpu_hand == -1:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの勝ち')
elif player_hand - cpu_hand == 1:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの負け')
elif player_hand - cpu_hand == -2:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの負け')
else:
print('例外エラー')
それでは各セクションごとのプログラムの内容を解説していきます。
import random
cpu_hand = random.randint(0,2)
最初のimport分でrandomライブラリを参照しますよと宣言をして
乱数を生成できる準備をしています。
準備が完了したら0~2までの整数値で乱数を発生させ、
発生させた乱数をcpu_handという変数に代入しています。
プログラム的にはここでCPUの手が決まってきます。
続いてplayerのじゃんけんの手を決めます。
player_hand = input('じゃんけんの手を入力してください:(0=グー、1=チョキ、2=パー)')
player_hand = int(player_hand)
input分で実際にどの手を出すのか入力をさせます。
入力された値はplayer_handという変数に格納されます。
注意したいのはplayer_handには入力された0~2の数字が入りますが
pythonが認識するのは整数の0~2ではなく
文字列としての0~2となりますので注意しましょう。
この後の条件式の中で整数値として扱いたいので整数値(int)に型を変換して
同じ名前の変数に代入をしています。
次にコンピュータとplayerのじゃんけんの手をif文で判定をして人間にわかるように
じゃんけんの手をcpu_resultとplayer_resultに文字列型で格納します。
if cpu_hand == 0:
cpu_result = 'グー'
elif cpu_hand == 1:
cpu_result = 'チョキ'
else:
cpu_result = 'パー'
if player_hand == 0:
player_result = 'グー'
elif player_hand == 1:
player_result = 'チョキ'
else:
player_result = 'パー'
次のif文はcpuとplayerのじゃんけんの手を比較して勝敗の判定を行っています。
表示の部分はfomat関数を利用していますがシンプルに描きたい場合はf文字列を利用するとシンプルに記載ができます。
if player_hand >= 3:
print('無効試合')
elif player_hand == cpu_hand:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あいこ')
elif player_hand - cpu_hand == 2:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの勝ち')
elif player_hand - cpu_hand == -1:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの勝ち')
elif player_hand - cpu_hand == 1:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの負け')
elif player_hand - cpu_hand == -2:
print('player:{}'.format(player_result))
print('cpu:{}'.format(cpu_result))
print('あなたの負け')
else:
print('例外エラー')
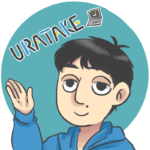
制御構文のif文についてご紹介させていただきました。
次回からは動作するプログラムをベースにプログラムの内容を紹介しながら
内容を理解できるような構成にしていこうと思います。
コメント